Comparing TestNG vs. JUnit: Which One Is Right for Your Project?
- Astalakshmi Amulraj
- Dec 19, 2024
- 4 min read
When deciding between TestNG and JUnit for your project, evaluate their features, capabilities, and use cases. Both are widely used testing frameworks in the Java ecosystem but cater to slightly different requirements.
TestNG:
Developed by Cédric Beust, TestNG (Test Next Generation) is an advanced testing framework and it is developed from JUnit but with additional functionalities like parallel execution, annotations, dependency testing, etc.
JUnit:
JUnit is a lightweight and widely used unit testing framework in Java and it is mainly for writing and running repeatable test cases.
Feature Comparison: TestNG vs. JUnit
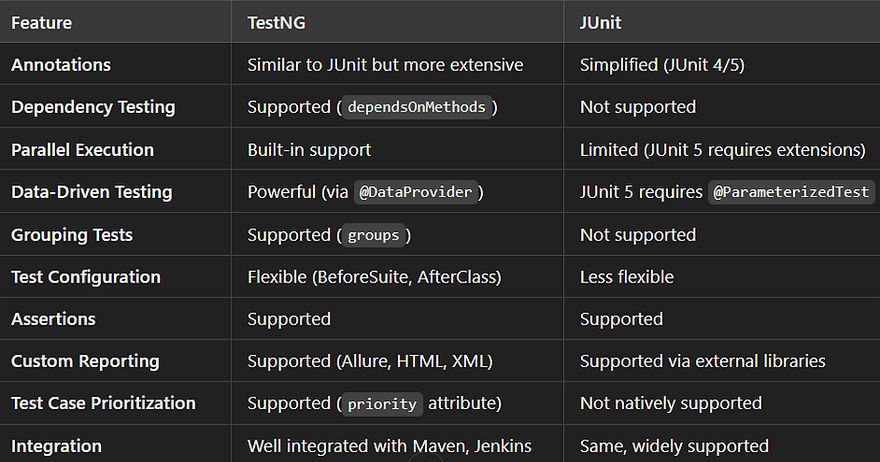
Model Execution
TestNG :
TestNG supports flexible execution models including grouping and prioritizing tests using various annotations like @Test(groups="groupName") and @Test(priority=n).
It also supports soft assertions, allowing execution to continue even after a test failure, which is useful for scenarios where we want to log multiple issues in a single test run.
JUnit:
JUnit is a more modular architecture that was introduced using the Jupiter engine, enhancing test discovery and execution. However, it still lacks support for test prioritization.
Annotations
TestNG :
TestNG uses annotations similar to JUnit but it offers additional options:
@BeforeSuite, @AfterSuite, @BeforeTest, @AfterTest for suite-level configurations.
@DataProvider for advanced data-driven testing.
@Test(enabled=false) allows test cases to be disabling.
Sample Program
import org.testng.annotations.*;
public class TestNGAnnotationsDemo {
@BeforeSuite
public void beforeSuite() {
System.out.println("Executed Before Suite - Setup configurations for the suite.");
}
@AfterSuite
public void afterSuite() {
System.out.println("Executed After Suite - Cleanup configurations for the suite.");
}
@BeforeTest
public void beforeTest() {
System.out.println("Executed Before Test - Prepare preconditions for the test.");
}
@AfterTest
public void afterTest() {
System.out.println("Executed After Test - Cleanup after the test.");
}
@DataProvider(name = "sampleDataProvider")
public Object[][] dataProvider() {
return new Object[][] {
{"Data1", 1},
{"Data2", 2}
};
}
@Test(dataProvider = "sampleDataProvider")
public void testWithDataProvider(String data, int number) {
System.out.println("Test with DataProvider executed with: " + data + " and " + number);
}
@Test(enabled = false)
public void disabledTest() {
System.out.println("This test is disabled and won't run.");
}
@Test
public void regularTest() {
System.out.println("Regular test case executed.");
}
}
Output:
JUnit :
JUnit uses cleaner annotations:
@BeforeEach, @AfterEach for test setup/teardown.
@Test for test methods.
Sample Program
import org.junit.jupiter.api.*;
public class JUnitAnnotationsDemo {
@BeforeEach
void setUp() {
System.out.println("Executed Before Each Test - Setting up preconditions.");
}
@AfterEach
void tearDown() {
System.out.println("Executed After Each Test - Cleaning up after test.");
}
@Test
void testExample1() {
System.out.println("Test Example 1 executed.");
Assertions.assertTrue(true, "This test passes.");
}
@Test
void testExample2() {
System.out.println("Test Example 2 executed.");
Assertions.assertEquals(5, 2 + 3, "Sum calculation should be correct.");
}
}
Output:
Dependency Testing
TestNG :
TestNG allows us to specify dependencies using the dependsOnMethods or dependsOnGroups attributes in @Test annotation between the methods.
This feature is useful when the execution of one test depends on the success of another.
JUnit:
JUnit does not support direct dependency management between test methods.
However, dependencies can still be handled programmatically or with careful structuring of the test cases.
Parallel Execution
TestNG :
Parallel execution is supported and configurable through an XML file.
This file is particularly beneficial in reducing test execution time for large-scale applications with multiple environments or browser configurations.
For example, Testing a web application across Chrome, Firefox, and Edge simultaneously is straightforward with TestNG.
TestNG allows us to execute tests in parallel by configuring the XML files.
JUnit:
Parallel execution is available in JUnit 5 but requires configuration with tools like Maven Surefire or Gradle.
It is less intuitive than TestNG but sufficient for smaller tests.
Data-Driven Testing
TestNG :
Uses @DataProvider to supply test data. It is flexible and supports custom methods returning data arrays.
Also, it is easier to handle complex or large datasets with multiple parameters. Data providers can be reused across test cases and classes in TestNG
With @DataProvider, TestNG makes it easy to provide multiple data sets and it is shown below.
JUnit:
Uses @ParameterizedTest with sources like @CsvSource and @ValueSource. It has Built-in support for CSV, files, and custom methods. Also, it is Simpler for lightweight, basic data-driven tests.
Grouping Test
TestNG :
TestNG allows explicit test grouping using the groups attribute in the @Test annotation.
These Tests can be assigned to multiple groups. and also specific groups can be included or excluded in the XML configuration file.
JUnit:
JUnit uses the @Tag annotation (introduced in JUnit 5) to perform group tests. Each test can be tagged with one or more tags. Using build tools like Maven or Gradle Specific tags can be included or excluded during execution.
Reporting
TestNG :
TestNG generates detailed HTML and XML reports.
It will also summarize test results, execution time, and skipped tests.
These reports are customizable and integrate easily with tools like Allure for visualization.
JUnit:
JUnit relies on third-party tools like Maven Surefire or Gradle for report generation.
While this adds flexibility, it may require more setup for reporting.
TestNG or JUnit?
TestNG ?:
If we need parallel execution for faster test runs.
If we require dependency management between test cases.
If we want powerful data-driven testing using @DataProvider.
If we need advanced features like test grouping, prioritization, and flexible reporting.
Example: End-to-end testing of a complex web application.
JUnit?:
If we are working on unit testing or lightweight testing projects.
If we prefer a simpler and more structured approach.
If we are using JUnit 5, which supports modern features like @ParameterizedTest and extensions.
If we require backward compatibility for legacy systems.
Example: Unit testing modules or microservices in a development project.
Conclusion

The projects with complex testing scenarios, such as end-to-end automation or dependency testing, TestNG is a better choice. However, if we focus on unit testing or a lightweight, clean testing approach, JUnit (especially JUnit 5) is a good choice.
Both frameworks are powerful, and a great choice depending on your project requirements and team familiarity.