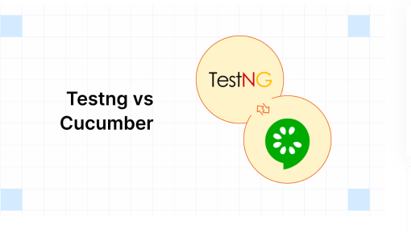
Introduction
When it comes to automation testing in Java, Cucumber and TestNG are two of the most widely used frameworks. While both serve the purpose of test execution, they cater to different testing needs.
Cucumber is designed for Behavior-Driven Development (BDD) and focuses on writing tests in a human-readable format.
TestNG is known for its flexibility in creating and executing test cases across various test suites and it provides powerful features for functional, integration, and unit testing.
1. What is Cucumber?
Cucumber is an open-source BDD (Behavior-Driven Development) framework that allows writing test cases in a simple, Gherkin syntax. It promotes collaboration between developers, testers, and business stakeholders.
Key Features of Cucumber:
Uses Gherkin language (Given, When, Then, And) to define test scenarios.
Supports Step Definitions written in Java, Python, etc.
Can integrate with Selenium, Appium, and RestAssured for UI and API testing.
Generates detailed test reports (Cucumber Reports, Extent Reports, Allure reports, cucumber failed reports ).
Supports data-driven testing using Examples tables in Gherkin.
Scenario: scenario is sequence of steps to test a specific functionality.
Given: steps are used to describe the initial context of the system - the scene of the scenario. It is typically something that happened in the past or Given is a precondition.
When: steps are used to describe an event, or an action or When I login to the site with a valid username and password (user action).
Then: steps are used to describe an expected outcome, or result.
The step definition of a Then step should use an assertion to compare the actual outcome (what the system actually does) to the expected outcome (what the step says the system is supposed to do).
And: success
Data-Driven Testing: Cucumber supports Data Driven Testing using Scenario Outline and Examples keywords. Creating a feature file with Scenario Outline and Example keywords helps to reduce the code and test multiple scenarios with different input and validation values.
Example Cucumber Feature File:
Feature: Login Functionality
Scenario: Login with valid credentials
Given The user is on the Login Page
When The user entering valid username and valid password
And The user clicks Login button
Then The user should redirected to register page
Step Definition in Java:
@Given("The user is on the LoginPage")
public void the_user_is_on_the_login_page() {
login.navigatetoLoginpage();
Assert.assertTrue(login.isOnLoginPage());
driver.get("https://example.com/login");
}
2. What is TestNG?
TestNG (Test Next Generation) is a powerful test automation framework inspired by JUnit but with additional features. It is commonly used for unit testing, functional testing, and regression testing.
Key Features of TestNG:
Uses annotations like @Test, @BeforeMethod, @AfterMethod, etc.
Supports parallel execution of test cases.
Has built-in support for data-driven testing (@DataProvider).
Generates detailed test reports (TestNG Reports, TestNG failed reports, Extent Reports).
Allows dependency testing and grouping of test cases.
@BeforeMethod: @BeforeMethod is executed before each test method within a test class.
example open the browser.
@Test: @Test test cases will executed.
@AfterMethod: @AfterMethod is executed after each test method within a test class.
example close the browser.
Parallel Execution: Parallel testing is a process where multiple tests are executed simultaneously(in parallel ).
@DataProvider: DataProvider enables data-driven testing, allowing the same test method to be executed multiple times with different sets of input data.
Grouping: grouping is allows you to organize and run specific sets of test cases together (e.g., Smoke, Regression, Sanity)..
Unit Testing: Unit testing is a checks single code pieces.
Functional Testing: Functional testing is a type of software testing that verifies that each function of the application operates according to the requirements.
Regression Testing: Regression testing ensures that a product’s final release functions as expected in the production environment. Regression testing verifies that new features or changes do not impact old functionality.
Example TestNG Test Case:
@Test(dataProvider = "loginDataProvider", dataProviderClass = DataProviders.class , groups="Dataprovider")
public void Logintest() {
login.clickGetStartedbutton();
login.SignInBtn();
3. Key Differences: Cucumber vs TestNG
Feature | Cucumber | TestNG |
Approach | BDD (Behavior-Driven) | TDD (Test-Driven) |
Test Definition | Written in Gherkin (natural language) | Written in Java (code-based) |
Best Suited For | Functional, UI, and API testing in BDD projects | Unit, Functional, and Integration Testing |
Annotations | Uses Gherkin (Given, When, Then) | Uses Java annotations (@Test, @BeforeMethod, etc.) |
Parallel Execution | Requires TestNG or JUnit integration | Built-in support for parallel execution |
Data-Driven Testing | Uses Examples table in Gherkin | Uses @DataProvider annotation |
Reports | Generates Cucumber Reports, Extent Reports | Generates TestNG Reports, Extent Reports |
Integration | Works well with Selenium, Appium, RestAssured | Works well with Selenium, Appium, API testing tools |
Re-running Failed Tests | Uses Rerun Plugin | Uses testng-failed.xml |
Readability | High (business users can understand) | Lower readability for non-technical users |
4.Cucumber and TestNG Work Together
Cucumber can be integrated with TestNG to utilize both the power of BDD and TestNG features like parallel execution and listeners.
This allows BDD tests to be executed in parallel using TestNG.
Example: Using Cucumber with TestNG Runner
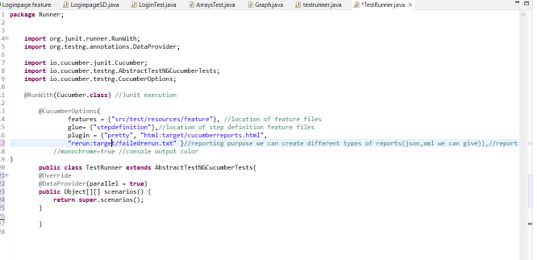
Conclusion
Both Cucumber and TestNG have their own strengths and are used in different contexts.
Cucumber is best for behavior-driven testing where test cases need to be written in a human-readable format.
TestNG is a robust test framework with powerful execution, assertion, and reporting features.
For many projects, we can use combination of both.